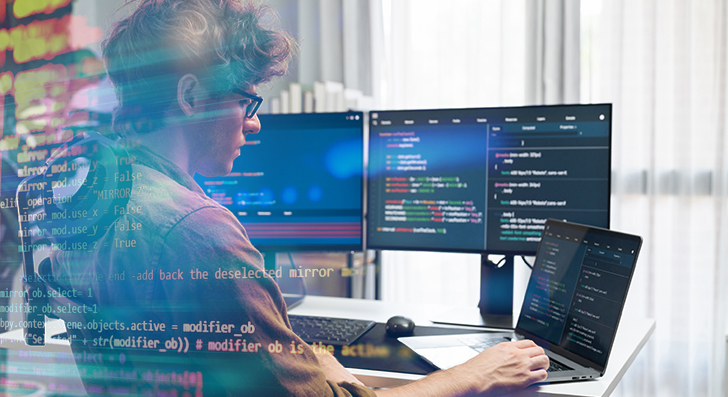
Scalability suggests your software can cope with expansion—a lot more customers, extra facts, and even more site visitors—without having breaking. As a developer, making with scalability in mind will save time and pressure later. Below’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't a thing you bolt on later—it should be portion of one's system from the beginning. Quite a few applications fall short once they improve quick because the initial design can’t take care of the additional load. As being a developer, you might want to Believe early regarding how your system will behave stressed.
Start by planning your architecture to be versatile. Prevent monolithic codebases exactly where almost everything is tightly related. Rather, use modular layout or microservices. These patterns split your application into lesser, independent areas. Each individual module or services can scale on its own devoid of influencing The entire process.
Also, think about your database from day one particular. Will it will need to take care of a million customers or maybe 100? Choose the correct sort—relational or NoSQL—determined by how your details will grow. Strategy for sharding, indexing, and backups early, even if you don’t want them nonetheless.
Another essential level is in order to avoid hardcoding assumptions. Don’t publish code that only will work underneath present-day disorders. Think about what would happen When your consumer base doubled tomorrow. Would your app crash? Would the database decelerate?
Use design styles that aid scaling, like information queues or celebration-pushed units. These assistance your application cope with additional requests devoid of receiving overloaded.
If you Create with scalability in mind, you're not just getting ready for success—you might be cutting down future head aches. A perfectly-prepared technique is simpler to take care of, adapt, and increase. It’s far better to organize early than to rebuild later.
Use the ideal Databases
Selecting the correct databases is often a vital Element of making scalable apps. Not all databases are developed the identical, and using the Incorrect you can sluggish you down or perhaps trigger failures as your app grows.
Get started by being familiar with your knowledge. Is it really structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a superb healthy. These are definitely sturdy with relationships, transactions, and regularity. Additionally they aid scaling tactics like study replicas, indexing, and partitioning to manage much more targeted visitors and knowledge.
When your data is much more adaptable—like consumer exercise logs, item catalogs, or files—contemplate a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing massive volumes of unstructured or semi-structured data and may scale horizontally extra effortlessly.
Also, look at your study and publish styles. Are you currently executing lots of reads with much less writes? Use caching and skim replicas. Have you been dealing with a major create load? Investigate databases which can deal with substantial produce throughput, or even celebration-centered information storage techniques like Apache Kafka (for momentary details streams).
It’s also smart to Believe forward. You may not will need Highly developed scaling features now, but picking a databases that supports them suggests you received’t need to switch later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your knowledge determined by your entry patterns. And always keep track of database overall performance as you develop.
In brief, the correct database is determined by your app’s structure, pace demands, And the way you count on it to expand. Get time to pick wisely—it’ll save loads of hassle afterwards.
Enhance Code and Queries
Speedy code is essential to scalability. As your application grows, each and every tiny delay provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s important to Establish efficient logic from the beginning.
Start off by creating clean, simple code. Stay clear of repeating logic and remove something unnecessary. Don’t pick the most sophisticated Answer if a simple one will work. Maintain your capabilities limited, targeted, and straightforward to test. Use profiling tools to search out bottlenecks—areas where your code can take far too extended to operate or employs an excessive amount of memory.
Future, examine your databases queries. These usually gradual factors down more than the code by itself. Make sure each query only asks for the info you actually will need. Steer clear of Pick out *, which fetches every little thing, and in its place pick precise fields. Use indexes to speed up lookups. And keep away from doing a lot of joins, especially across significant tables.
In the event you detect the same info remaining requested many times, use caching. Shop the outcome quickly using applications like Redis or Memcached so that you don’t really need to repeat highly-priced functions.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and helps make your application additional economical.
Make sure to take a look at with significant datasets. Code and queries that function fantastic with one hundred data could crash every time they have to handle 1 million.
In short, scalable apps are quickly apps. Keep the code limited, your queries lean, and use caching when desired. These steps help your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle more users and much more targeted traffic. If anything goes by just one server, it can promptly turn into a bottleneck. That’s the place load balancing and caching can be found in. These two resources assist keep your application speedy, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout many servers. As an alternative to one particular server carrying out each of the function, the load balancer routes users to distinctive servers dependant on availability. What this means is no solitary server gets overloaded. If a person server goes down, the load balancer can send out visitors to the Other individuals. Resources like Nginx, HAProxy, or cloud-based alternatives from AWS and Google Cloud make this simple to setup.
Caching is about storing details briefly so it may be reused quickly. When people request the same facts once again—like a product page or maybe a profile—you don’t must fetch it from the databases each time. You could serve it from the cache.
There are 2 typical sorts of caching:
1. Server-facet caching (like Redis or Memcached) shops details in memory for quickly accessibility.
two. Client-aspect caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching cuts down database load, increases speed, and would make your app additional effective.
Use caching for things which don’t modify normally. And often be certain your cache is up to date when facts does alter.
Briefly, load balancing and caching are simple but effective applications. With each other, they help your application handle a lot more buyers, stay rapidly, and Get better from troubles. If you propose to grow, you will need both equally.
Use Cloud and Container Tools
To construct scalable apps, you would like tools that let your app increase conveniently. That’s where cloud platforms and containers are available in. They offer you adaptability, decrease setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to obtain components or guess long run potential. When targeted visitors increases, you'll be able to incorporate far more methods with just a couple clicks or mechanically working with car-scaling. When website traffic drops, you may scale down to economize.
These platforms also present expert services like managed databases, storage, load balancing, and protection equipment. It is possible to target constructing your app rather than handling infrastructure.
Containers are An additional important tool. A container offers your application and almost everything it has to run—code, libraries, configurations—into just get more info one unit. This makes it easy to maneuver your application among environments, from your notebook on the cloud, without having surprises. Docker is the most well-liked Instrument for this.
When your application makes use of numerous containers, applications like Kubernetes make it easier to control them. Kubernetes handles deployment, scaling, and recovery. If just one element of your application crashes, it restarts it instantly.
Containers also make it very easy to independent aspects of your app into services. You can update or scale sections independently, which can be perfect for functionality and reliability.
Briefly, utilizing cloud and container instruments indicates you could scale quickly, deploy easily, and Recuperate immediately when difficulties materialize. If you need your application to expand without the need of limitations, start employing these applications early. They conserve time, lower danger, and allow you to continue to be focused on constructing, not correcting.
Keep track of Anything
If you don’t check your software, you received’t know when things go Mistaken. Checking allows you see how your app is undertaking, spot concerns early, and make superior conclusions as your app grows. It’s a crucial Section of setting up scalable systems.
Commence by tracking primary metrics like CPU use, memory, disk House, and response time. These tell you how your servers and providers are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this information.
Don’t just check your servers—check your application much too. Keep an eye on how long it takes for customers to load pages, how often mistakes occur, and in which they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Setup alerts for essential issues. As an example, In case your response time goes above a Restrict or simply a company goes down, you'll want to get notified immediately. This helps you take care of challenges rapid, typically ahead of consumers even discover.
Monitoring is usually handy if you make adjustments. In the event you deploy a new aspect and find out a spike in mistakes or slowdowns, you can roll it again ahead of it leads to serious problems.
As your app grows, traffic and facts raise. With no monitoring, you’ll miss out on signs of hassle right up until it’s as well late. But with the right instruments in place, you keep in control.
To put it briefly, monitoring allows you maintain your application trustworthy and scalable. It’s not just about spotting failures—it’s about understanding your technique and making sure it really works well, even stressed.
Final Feelings
Scalability isn’t only for major businesses. Even smaller apps need to have a strong foundation. By building very carefully, optimizing sensibly, and using the appropriate tools, it is possible to Establish apps that increase effortlessly without having breaking stressed. Start tiny, Imagine large, and Create good.